If you would like to review the previous posts in this series, please feel free to review the links below:
- Starting with jQuery
- Starting with jQuery - Effects
- Starting with jQuery - Intro to Validation Plug-in
- Starting with jQuery - Dynamically Applying Rules
Required Downloads to use the Examples:
In order to use the Validation plug-in, you'll need to add a few things to your web page:- jQuery (version 1.2.6 is used in this example)
- jQuery Validation Plug-In
Code Downloads for this Post:
Source Code Download: Starting With jQuery - Custom Validation Rules.zipJust the Rules File: Starting With jQuery - Additional Validation Rules.zip
A Look at some of the Built-In Validation Rules:
A wiser man than I once said that you should strive to never be your own plumber in software development. Ultimately, you shouldn't spend time writing something that has already been written for you by someone else, is accessible, and meets your needs. To go along with this concept I think it would be best to briefly cover what rules are already built into this excellent plug in.- Required: The typical and probably most used validation out there.
- Minlength & Maxlength: Staple Length validation rules
- Rangelength: Similar to Min/Maxlength; allows for selected item counts as well.
- Min & Max & Range: Same as the "length" rules above only for numeric values.
- Email: A very robust email validation rule
- Url: Ensures the input is a well formatted url.
- Date: Verifies the input can be parsed into a date.
- Number: Ensures that the input is numeric.
- Digits: Ensures only 0-9 characters (no commas, decimal points, etc.)
- Credit Cards: Another complex and common validation rule made easy.
- Accept: A file extension validation rule.
- EqualTo: Ensures that the values of 2 elements are the same.
Creating a Custom Validation Rule:
Now that we know what's already available, there may come a situation where we find that the built in rules just don't cover everything we need. When such an occurrence comes upon us, we have the ability to extend the Validation Plug-in. Custom validation rules are, ultimately, methods that are called and return a boolean value; true if the test succeeds and false if the test fails. After the methods are created, they can be wired up to the jQuery validation component and used like any other rule.To create a function that will encapsulate our validation test, we need to use one of the following signatures:
function myTest(value, element){...}
//OR
function mytest(value, element, params){...}
These function signatures, are identical except for the last parameter. The value parameter houses the value that will be tested. The element represents the element that is being tested. The params involves any data that is being passed into the test (i.e. a minimum length value).
Looking over the list of built in validation rules, I noticed that one that was missing was a "Text Only" rule to ensure that only alpha characters would appear in the field. Using this as an example, our validation rule function would look something like this:
function myTextOnlyValidation(value, element){
return /[a-zA-Z ]/.test(value);
}
After we have the function established, we can then attach it to the jQuery Validation plug-in. To do this, we call the validator object's addMethod() function. This addMethod() function takes 3 parameters; a label for the rule, the function that contains the test, and the default message to display when the test fails.
$.validator.addMethod("textOnly", myTextOnlyValidation, "Please enter only alpha characters( a-z ).");
Now that the function has been added, we can use the rule, called "textOnly", just like any of the built in rules.
Putting it all Together:
Now that we've looked at the built in rules, created our own rule, and wired up our custom rule; all that's left is to put everything together. In this example, we're going to keep things simple and use just a single text box, a label, and a submit button (see Figure 1 below).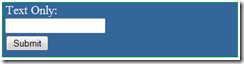
Figure 1 - the default form
For the example, we'll be applying 2 validation rules to this textbox. The first will be the same required rule that we applied when we were first introduced to the Validation Plug-in. The second rule will be our custom textOnly rule. In order to do such, we need to add the following JavaScript to our code:
1: //Our validation script will go here.
2: $(document).ready(function(){
3:
4: //custom validation rule - text only
5: $.validator.addMethod("textOnly",
6: function(value, element) {
7: return !/[0-9]*/.test(value);
8: },
9: "Alpha Characters Only."
10: );
11:
12: //validation implementation will go here.
13: $("#TestForm").validate({
14: rules: {
15: txtTextOnly: {
16: required: true,
17: textOnly: true
18: }
19: },
20: messages: {
21: txtTextOnly: {
22: required: "* Required"
23: }
24: }
25: });
26: })
After the JavaScript has been applied to the form, we can verify that it works, as shown in Figure 2.

Figure 2 - Validating the Custom Rule
Some Additional Custom Rules:
I used the TextOnly rule in the example because it's a rather simple one to illustrate; however, any number of rules can be applied in the same way. In order to provide additional content and to hopefully give others a jumpstart on implementing the jQuery Validation Plug-in onto their site, I have also added some additional custom rules. These additional rules, available with in the example site or as a separate download, provide the following functionality:- Phone Numbers (in ###-###-#### format)
- US Postal Codes ( ##### or #####-#### format)
- Canadian Postal Codes
UPDATE 25 September 2012:
Since this is one of my most popular posts (THANK YOU!) I decided to address some comments about the example I wrote 4 years ago not working today.
In terms of the advances of jQuery and the jQuery validation plugin, the code sample will still work properly; however, you'll need to upgrade both of them to the most recent versions (as of this update jQuery 1.8.2 and jQuery Validation 1.9). Once this is done, the code sample will function continue to function as designed.
There is 1 small bug in the code sample though. The textOnly rule found in ValidationRules.js and AdditionalRules.js has a bug with the regular expression. Instead of an asterisk (*) in the regular expression pattern, it should be a plus-sign (+) to signify 1 or more instead of 0 or more. Correcting this little bug will allow you to use the example rules without an issue.
Awesome article. Thanks! I was able to write a custom validation rule that checks to see if a file has a csv extension. Can you give a quick example code showing how to write a rule that does not use a regex? For example, how can I write a custom rule that checks to see if at least one checkbox in a group is checked?
ReplyDeleteThank you. Just what I needed.
ReplyDeleteEd, there's a built-in rule for validating extensions. I think it's called... "extension".
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteThanks man... Your example of a custom validation rule is very clear and made it quite easy to implement.
ReplyDeleteEvery other example I saw (looked at about 7-8 pages before I found yours) was way too complex, verbose, cumbersome or something else.
Thanks for the simplicity and clarity.
Thank you very much for this article on custom jQuery validation. This was the first example I have used that actually worked. Others I have seen leave out code that is essential to the validator working. Much props!!
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteAnybody know how to compare two dates and validate that the first is less than the second, i got a problem with this, i tried validate building date objects, spliting and checking every term(day,month,year), and by any reason i got always true or false, any help?
ReplyDeleteThanks
ReplyDeleteThanks a lot
ReplyDeletehi there, i have a question, how can i validate two date inputs on a text input, the second date on the text box should be a higher date. i need to doit with validation.js can you helpme
ReplyDeleteThanks for the tutorial. Very simple and easy to read and impliment. Only one question. How to color code the error messages something other than default black?
ReplyDelete@John
ReplyDeleteHi John,
To answer your question about changing the color of the error message, the Validation plug-in sets a css class on the error text. In order to change the color, all you have to do is add a CSS definition for the "error" class. This should take of it for you.
Hope this helps.
Hi James,
ReplyDeleteGreat blog post! I was looking everywhere for an easy to understand implementation of custom rules and yours was the best i found!
I got yours working but I'm having a little trouble implementing my own rule....
$.validator.addMethod("validTotalValue",
function(value) {
var outcome = parseInt($("#valuePerNight").val()) * parseInt($("#numberOfNights").val()) + parseInt($("#otherCharges").val()) != parseInt($("#totalValue").val())
alert("outcome:" + outcome)
return outcome;
},
"enter a valid totalValue"
);
I tested the logic and it works (outputs true/flase) I'm just not sure why its not hooking up and it doesn't highlight the error?
You have to use your method as a rule when you call .validate()
DeleteThanks a ton it was a wonderful help, now to write custom validation rules is simple and easy by using your advice. Thanks
ReplyDeleteExcellent introduction to customize jquery validator plugin.
ReplyDeleteNice Article. 100% Helpful and success effort. A+++++++
ReplyDeleteThanks~!
ReplyDeletereally nice..... Thanks
ReplyDeletei run the code above, but didn't work, am i lacking something?
ReplyDelete@marjune I just tested by pulling down the "Starting with jQuery - Validation Rules" zip file, opened default.htm in a browser (Chrome 21) and it worked properly. The example was written 4 years ago so I cannot guarantee that it works flawlessly with the most recent versions of jQuery and the jQuery validation plugin.
ReplyDelete@marjune I reviewed the code and was able to get it to function 100% with the latest versions of jQuery (1.8.2) and the jQuery validation plugin (1.9). I had to update both of them, though. I also found a bug with the textOnly rule which I have commented on in an update I have made to the blog post.
ReplyDeleteIf you are still having issues, please let me know and we'll figure out how to work through it offline perhaps.
Thanks for this tutorial
ReplyDeleteThis was very helpful! Many thanks!
ReplyDeleteAwesome article, thanks a lot...
ReplyDeleteHi James Eggers,
ReplyDeleteIm neal.
How will I validate if i want that users will not enter zero.
nice article ..
ReplyDeleteHi James Eggers,
is this possible if i want to have two or more textfield with same rules and message, but have different name of each textfield, or i must write rules and message of each textfield, thanks.
For each field, you'd need to list them separately in the rules section of the validation configuration.
DeleteFor example
$("#TestForm").validate({
rules: {
txtTextOnly: {required: true,textOnly: true},
txtTextOnly2: {required: true,textOnly: true}
},
messages: {
txtTextOnly: {required: "* Required"},
txtTextOnly2: {required: "* Required"}
}
});
Hope this helps
Thanks - a big help. It's a shame the author of the plugin wasn't as clear and as comprehensive as you have been.
ReplyDeletewhat if i have to check all the fields at once.
ReplyDeleteI dont want to write this long code
Great post! Couldn't figure it out from the JQuery docs - Thanks!
ReplyDeleteThanks! Great Post - very clear as usual
ReplyDelete